Adding Data in Vue.js: Dynamic Interpolation with {{}}
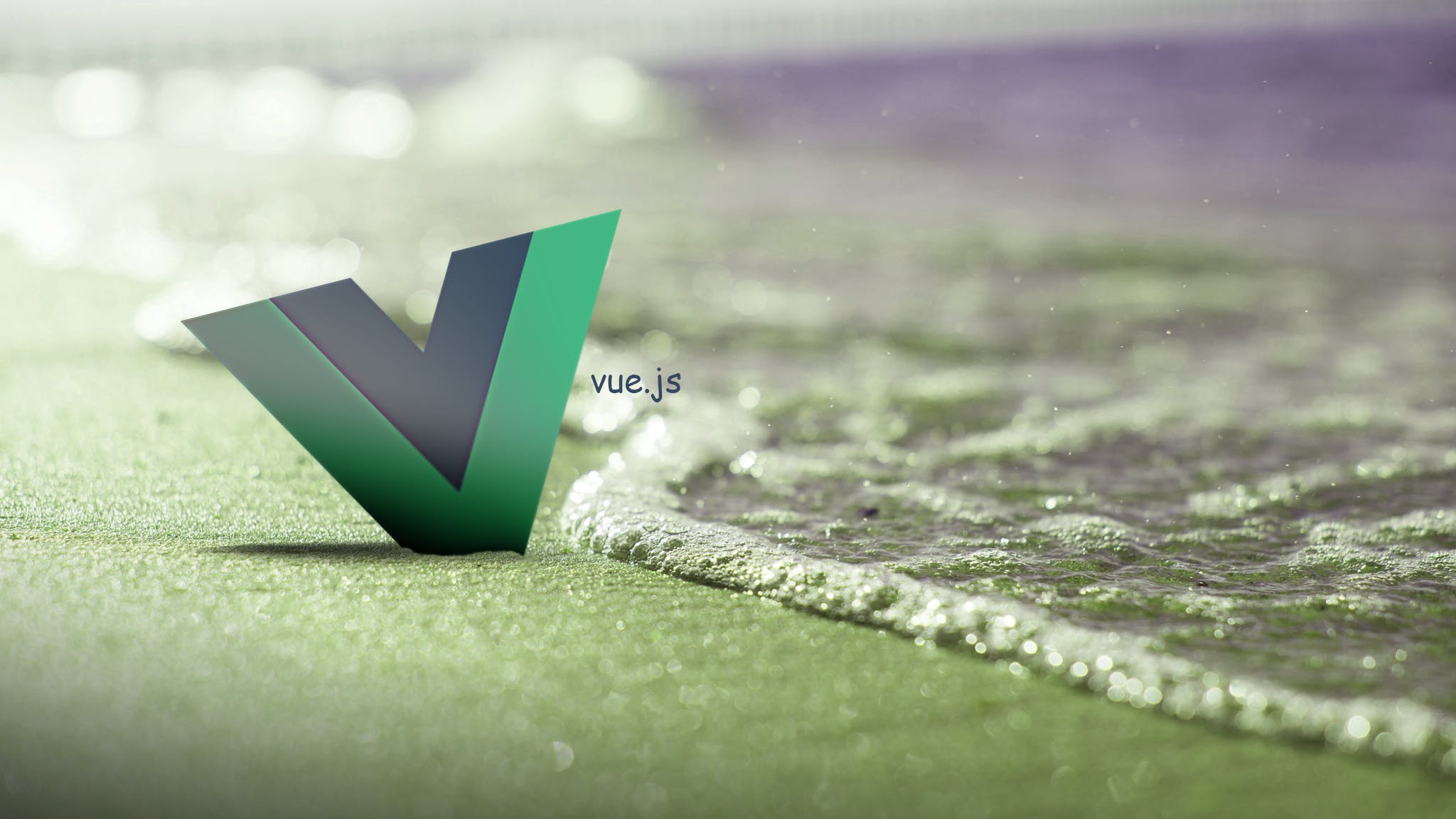
Simple Interpolation
Let's consider a basic example:
HTML
<div id="app">
<h1>{{ name }}</h1>
</div>
JavaScript
const app = Vue.createApp({
data() {
return {
name: "Alice Smith"
}
}
});
app.mount("#app");
Complex Interpolation
Interpolations can be used to display the results of more complex JavaScript expressions:
HTML
<div id="app">
<h1>{{ name + " " + surname }}</h1>
<p>Welcome, {{ greet(name) }}!</p>
</div>
JavaScript
const app = Vue.createApp({
data() {
return {
name: "Alice",
surname: "Smith"
}
},
methods: {
greet(name) {
return "Hello, " + name + "!";
}
}
});
app.mount("#app");
Use the code with caution.
Data Defined in createApp
Interpolations can access properties directly defined in the createApp function:
HTML
<div id="app">
<h1>{{ message }}</h1>
</div>
Use the code with caution.
JavaScript
const app = Vue.createApp({
data: {
message: "Welcome!"
}
});
app.mount("#app");
Use the code with caution.
Conclusion
Interpolation with {{}} provides an efficient way to integrate dynamic data into your Vue.js interface. It allows you to display variable values, execute complex expressions, and create interactive interfaces.
Bonus:
- Explore Vue.js directives to conditionally render elements or dynamically modify their styles.
- Refer to the official Vue.js documentation for a detailed explanation of interpolators and other features: https://vuejs.org/
Post a Comment
0Comments