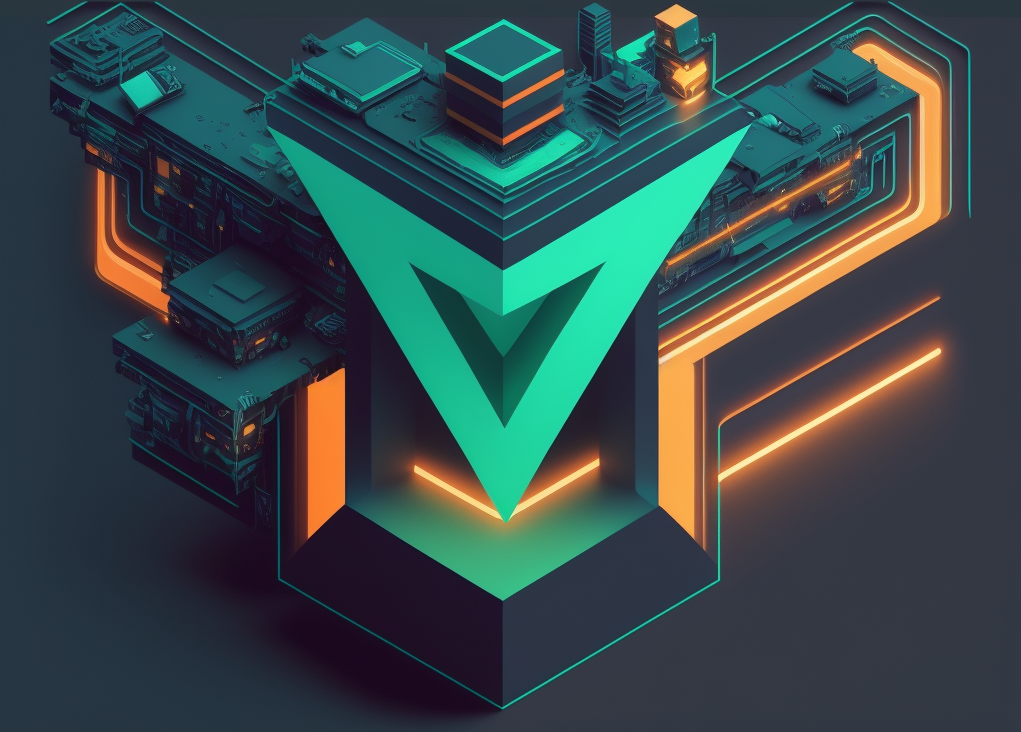
Exploring Vue Methods: Utilizing 'this' and Avoiding Proxies
Vue.js provides a powerful mechanism for defining methods within your Vue instances. While it's common to use arrow functions for method definitions, this can lead to unexpected behavior when dealing with 'this' context and proxy objects. Let's explore how to use conventional function syntax for Vue methods to ensure proper functioning and avoid pitfalls.
Using Conventional Function Syntax
Instead of using arrow functions, which maintain the lexical scope of 'this', Vue methods should be defined using the conventional function syntax. This allows Vue to bind the correct 'this' context, ensuring that methods can access data and other instance properties correctly.
Conventional function syntax looks like this:
methods: {
greet: function() {
// Method logic here
}
}
Avoiding Proxies
Vue instances internally use proxies to handle reactivity. When arrow functions are used for method definitions, Vue cannot properly proxy 'this', leading to unexpected behavior and potential bugs. By using conventional function syntax, Vue can accurately proxy 'this', ensuring reactivity works as expected.
Using arrow functions:
methods: {
greet: () => {
// Method logic here
}
}
Example
Here's an example demonstrating the use of conventional function syntax for Vue methods:
const app = Vue.createApp({
data() {
return {
message: 'Hello Vue!'
}
},
methods: {
greet: function() {
alert(this.message);
}
}
});
app.mount('#app');
Conclusion
By utilizing conventional function syntax for Vue methods and avoiding arrow functions, you can ensure proper 'this' context binding and avoid issues related to proxies. This approach leads to more predictable behavior and enhances the stability and reliability of your Vue.js applications.
Post a Comment
0Comments