Utilizing Proxy Data for Cleaner Vue.js Code
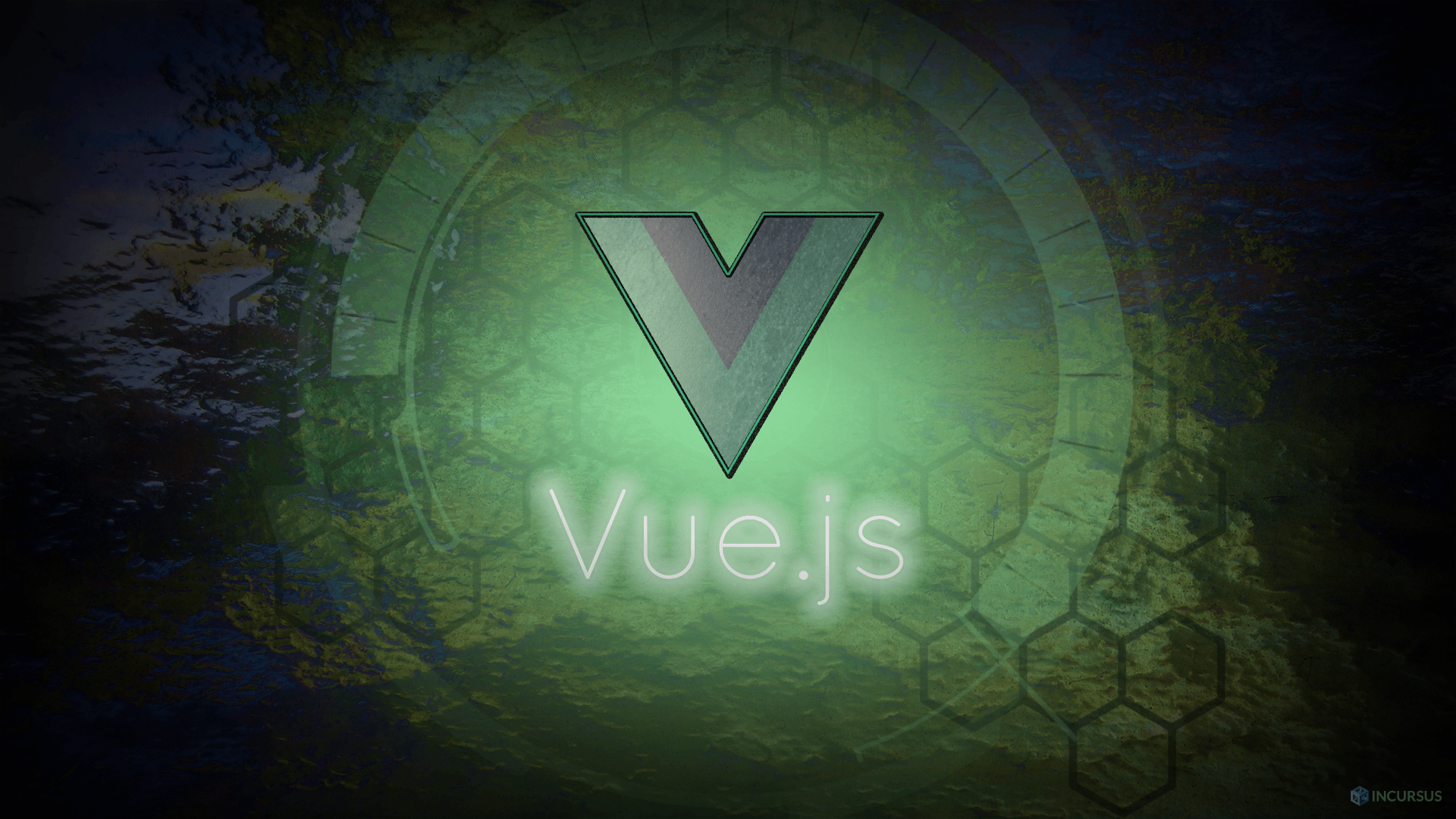
Vue.js is a powerful JavaScript framework renowned for its simplicity and efficiency in building user interfaces. One of its core features is reactivity, which enables automatic updating of the DOM when the underlying data changes. However, managing and mutating data in Vue.js can sometimes lead to verbose and error-prone code. Enter Proxy, a feature introduced in ES6, which Vue.js leverages to streamline data manipulation and enhance code readability.
In this article, we'll explore how to leverage Proxy data to make your Vue.js code cleaner and more maintainable. We'll also demonstrate how encapsulating Vue instance variables in a proxy can contribute to code organization and clarity.
Implementing Proxy Data in Vue.js
const app = Vue.createApp({
data() {
return {
user: {
name: 'John Doe',
age: 30,
email: 'john@example.com'
}
};
},
mounted() {
// Encapsulate data within a Proxy
this.$data.user = new Proxy(this.$data.user, {
set(target, key, value) {
console.log(`Setting ${key} to ${value}`);
target[key] = value;
return true;
}
});
}
});
// Mount the Vue app
const vm = app.mount('#app');
// Update user data using Proxy
vm.user.name = 'Jane Doe';
vm.user.age = 35;
Advantages of Using Proxy Data in Vue.js
- Enhanced Reactivity
- Code Clarity
- Improved Maintainability
Conclusion
Proxy data offers a powerful mechanism for managing and manipulating reactive data in Vue.js applications. By encapsulating Vue instance variables within Proxies, you can write cleaner, more maintainable code while enhancing reactivity and code clarity.
Consider incorporating Proxy data into your Vue.js projects to streamline data management and improve code organization. With Proxy, you'll unlock new possibilities for creating robust and efficient Vue.js applications.
Post a Comment
0Comments