The Evolution of Modularization in JavaScript: From Module.exports to Import/Export
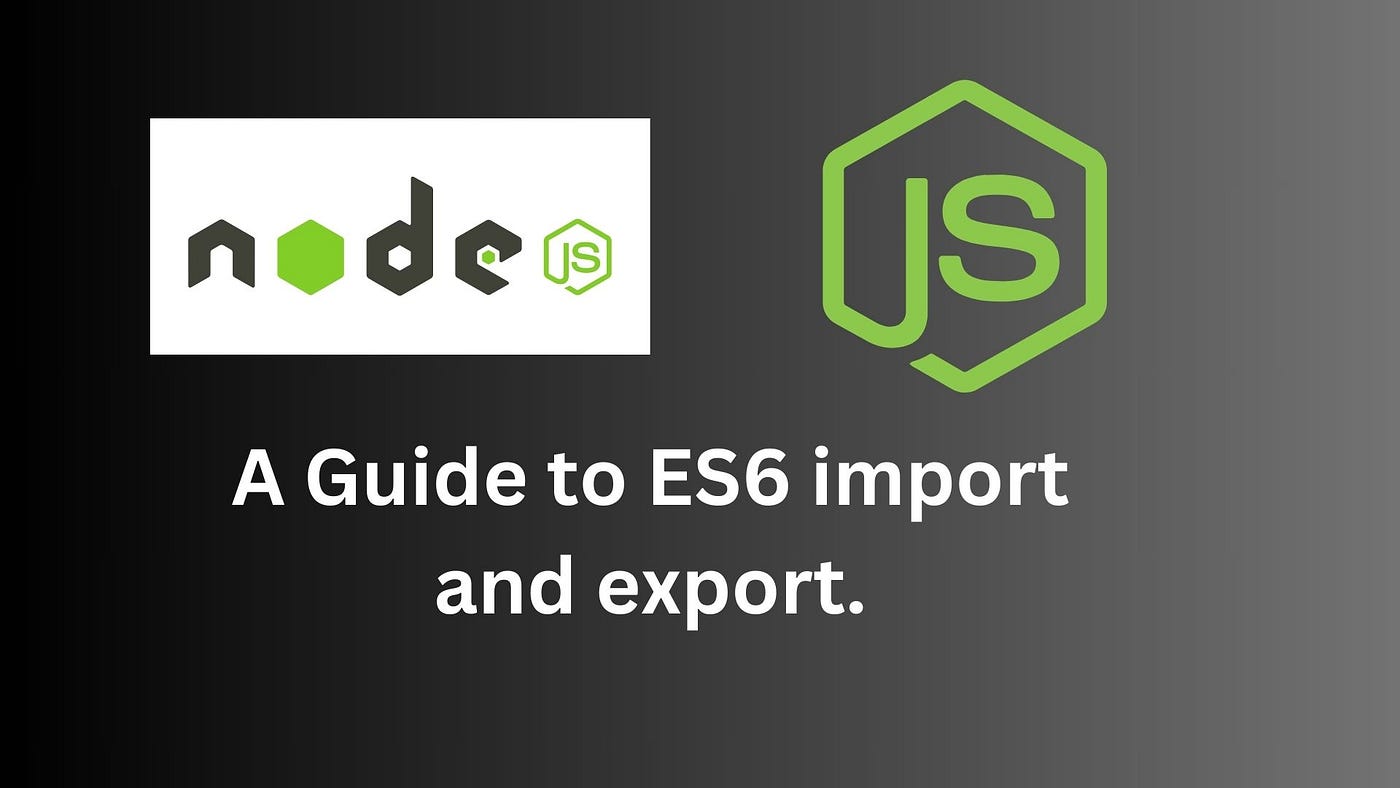
In the world of software development, modularization is an essential concept for managing and organizing code. In JavaScript, modularization methods have evolved considerably over time, providing developers with more options and flexibility in managing dependencies and project structure.
To understand this evolution, let's take a look at a simple script that calculates the sum of two numbers and displays the current directory:
// script.js
const a = 5;
const b = 4;
setTimeout(() => {
console.log(a + b);
console.log(__dirname);
}, 3000);
In the initial version of modularization in JavaScript, methods like module.exports
and require
were used to divide the code into reusable modules. Our script would have looked like this:
// Old version
const largeNumber = require('./script.js');
module.exports = { largeNumber: largeNumber };
In the new version of modularization, we use import/export
to achieve the same functionalities, but with more clarity and flexibility:
// New version
// script.js
const a = 5;
const b = 4;
setTimeout(() => {
console.log(a + b);
console.log(__dirname);
}, 3000);
const largeNumber = 356;
export { largeNumber };
// Node script.js
import { largeNumber } from './script.js';
By using the import/export
syntax, the code becomes clearer and easier to manage. It also provides greater control over imports and exports, allowing developers to import only what they need and better manage dependencies.
In conclusion, the evolution of modularization in JavaScript, from module.exports/require
to import/export
, has brought significant benefits in managing code and dependencies in modern JavaScript projects. This change not only makes the code clearer and easier to understand, but also offers greater flexibility and control to developers.
Post a Comment
0Comments