Using ES6 Modules in Node.js
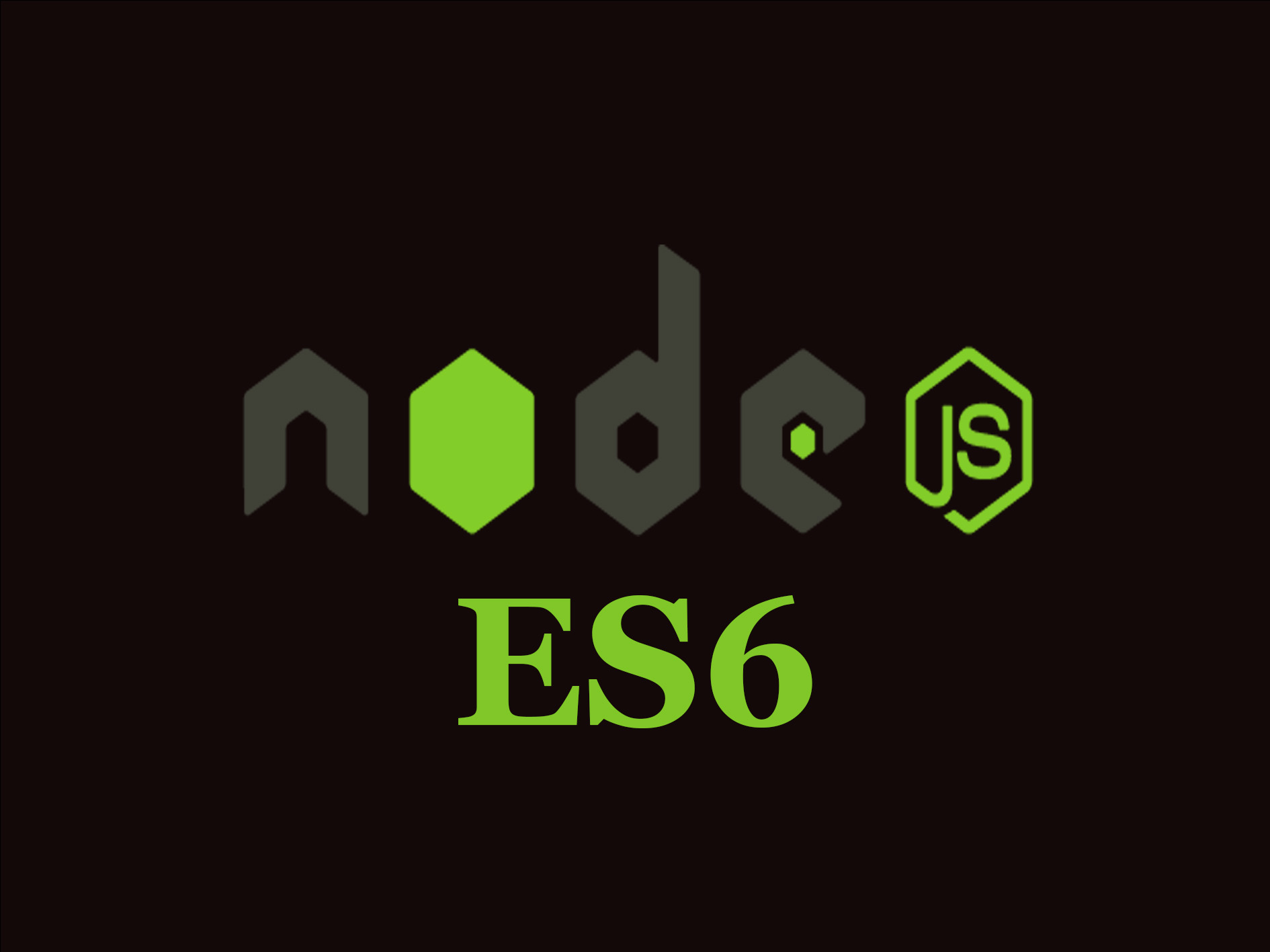
In a world of software development, change is inevitable and often necessary to stay relevant and efficient. One such significant change in the Node.js ecosystem is the adoption of ES6 modules as a modern and more flexible alternative to the traditional 'require' method. In this article, we will explore the importance and usage of ES6 modules in Node.js, along with some practical examples to illustrate the concepts.
Why ES6 Modules?
Prior to the advent of ES6 modules, Node.js used the 'require' method for loading modules and dependencies. However, this method was not fully compliant with ECMAScript specifications and had certain limitations. With the addition of ES6 module support in Node.js, developers gained access to a broader set of features and a clearer, more concise syntax.
Import Export Replaces 'require'
One of the most noticeable differences between ES6 modules and the traditional 'require' method is the use of 'import' and 'export' statements. Here's how you can import and export modules using ES6:
// Export from a file named script.js
// script.js
export const largeNumber = 1000;
// Import in another file
// another-file.js
import { largeNumber } from './script.js';
console.log(largeNumber); // will output: 1000
This syntax is clearer and more intuitive, making the code easier to read and maintain.
Using Destructuring
Another powerful feature of ES6 modules is the ability to use destructuring to extract only certain elements from imported modules. Here's how you can do it:
// Importing only largeNumber from script.js using destructuring
import { largeNumber } from './script.js';
console.log(largeNumber); // will output: 1000
Destructuring makes the code more elegant and efficient, allowing you to import only what you need from a module, thus reducing code complexity and size.
Setting up a Node.js Project with ES6 Modules
To start using ES6 modules in Node.js projects, it's important to ensure that the version of Node.js you're using supports this functionality. You can check the Node.js version using the 'node -v' command.
Additionally, to create a new Node.js project and initialize a 'package.json' file, you can use the 'npm init -y' command. This will create a default 'package.json' file, which will contain information about your project and its dependencies.
Conclusion
ES6 modules represent a significant evolution in the Node.js ecosystem, providing developers with a more elegant and powerful way to organize and share code. Through clearly defined import and export statements, along with the use of destructuring, developers can create applications that are easier to understand and maintain. With a simple setup using 'npm' and a solid understanding of ES6 module syntax, you can start benefiting from these enhanced features in your Node.js projects.
Post a Comment
0Comments